
A Deep Dive into Laravel for Beginners
A comprehensive guide for beginners who want to learn Laravel, covering everything from installation to building a simple application.
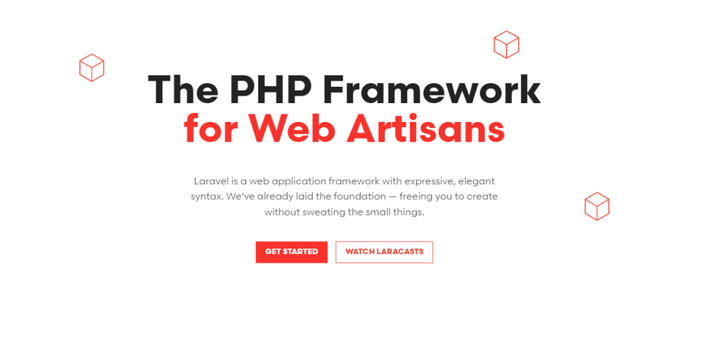
A Comprehensive Guide for Beginners to Learn Laravel
Laravel is a powerful PHP framework that simplifies the process of web application development. It’s known for its elegant syntax and developer-friendly tools. This guide will walk you through the essentials of Laravel, from installation to building your first simple application.
1. What is Laravel?
Laravel is a free, open-source PHP web framework designed for building modern, maintainable web applications. It follows the MVC (Model-View-Controller) architecture, which separates the application logic, presentation, and data.
2. Prerequisites to Learn Laravel
Before diving into Laravel, you should have:
- Basic knowledge of PHP: Understanding PHP syntax and basic programming concepts is essential.
- Basic knowledge of HTML, CSS, and JavaScript: Laravel involves rendering views with HTML, using CSS for design, and JavaScript for interactivity.
- Database knowledge (MySQL): Laravel uses databases for storing data, so basic knowledge of databases and SQL queries is important.
3. Setting Up Your Development Environment
To start developing with Laravel, you’ll need to set up your development environment.
Step 1: Install Composer
Laravel relies on Composer, a PHP dependency manager, to install and manage packages.
- Download Composer from here.
- Install Composer globally so it can be used from the command line.
Step 2: Install Laravel
Once Composer is installed, open your terminal and run:
composer global require laravel/installer
This command installs the Laravel installer globally, allowing you to create new Laravel projects easily.
Step 3: Create a New Laravel Project
To create a new Laravel project, run the following command:
laravel new my-app
Replace my-app
with the name of your application. Laravel will create a new project folder with the necessary files and dependencies.
Step 4: Set Up Your Local Development Server
Laravel comes with a built-in server for development. To start the server, navigate to your project directory and run:
php artisan serve
Your app will be accessible at http://127.0.0.1:8000
.
4. Understanding the Folder Structure
Laravel has a well-organized folder structure that makes it easy to navigate and maintain projects. Here are some key directories and files:
- app/: Contains the core business logic, controllers, models, etc.
- resources/views/: Contains your HTML templates (Blade templates).
- routes/web.php: Contains routes for your application.
- public/: The public-facing directory, which contains the
index.php
file and assets like images, CSS, and JavaScript.
5. Routes and Controllers
In Laravel, routes define the URL structure of your application, while controllers handle the logic for each route.
Step 1: Define a Route
Open routes/web.php
and define a basic route like this:
Route::get('/', function () {
return view('welcome');
});
This route points to the home page (/
), and it returns the welcome
view.
Step 2: Create a Controller
You can create a controller using the Artisan command:
php artisan make:controller HomeController
Then, define a method in the controller:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HomeController extends Controller
{
public function index()
{
return view('home');
}
}
Step 3: Use the Controller in Routes
Now, update the route to use the controller:
Route::get('/', [HomeController::class, 'index']);
This sets up the application to use the HomeController@index
method for the home route.
6. Blade Templates
Blade is Laravel's templating engine. It allows you to work with PHP in your HTML views.
Step 1: Create a Blade View
Create a new file in resources/views/
named home.blade.php
:
<!DOCTYPE html>
<html>
<head>
<title>Laravel App</title>
</head>
<body>
<h1>Welcome to Laravel!</h1>
</body>
</html>
This view will be rendered when the home route is accessed.
7. Database and Eloquent ORM
Laravel provides Eloquent, an ORM (Object Relational Mapping) system, to interact with databases. It allows you to work with database records as objects.
Step 1: Set Up the Database
In your .env
file, configure the database connection:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=root
DB_PASSWORD=
Step 2: Create a Migration
Migrations are like version control for your database schema. To create a migration for a posts
table, run:
php artisan make:migration create_posts_table
This will create a migration file in database/migrations/
.
Step 3: Define the Migration
Open the migration file and define the table schema:
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
Step 4: Run the Migration
Run the migration to create the table in the database:
php artisan migrate
Step 5: Create a Model
Models represent the database tables in Laravel. To create a model for the Post
table, run:
php artisan make:model Post
Now, you can interact with the posts
table using Eloquent methods, like:
$posts = Post::all();
8. Building a Simple CRUD Application
A simple "Create, Read, Update, Delete" (CRUD) application is a great way to practice Laravel.
Step 1: Create a Controller for CRUD
Generate a controller for managing posts:
php artisan make:controller PostController
Step 2: Define CRUD Methods
In PostController.php
, add methods for creating, reading, updating, and deleting posts:
public function create()
{
return view('create');
}
public function store(Request $request)
{
$post = new Post();
$post->title = $request->title;
$post->content = $request->content;
$post->save();
return redirect('/');
}
public function edit($id)
{
$post = Post::findOrFail($id);
return view('edit', compact('post'));
}
public function update(Request $request, $id)
{
$post = Post::findOrFail($id);
$post->title = $request->title;
$post->content = $request->content;
$post->save();
return redirect('/');
}
public function destroy($id)
{
Post::destroy($id);
return redirect('/');
}
Step 3: Define Routes for CRUD
In routes/web.php
, define the routes for each action:
Route::resource('posts', PostController::class);
This single line creates routes for all CRUD operations.
9. Conclusion
Congratulations! You’ve now gone through the basics of installing Laravel, setting up routes, creating controllers, and interacting with the database using Eloquent. From here, you can expand your knowledge by learning about:
- Authentication (using Laravel’s built-in tools)
- Middleware
- Laravel Mix (for compiling assets)
- Testing with PHPUnit
Laravel has an extensive documentation that can guide you through more advanced topics. Keep practicing and building projects to solidify your understanding!
Share this post:
Related Posts
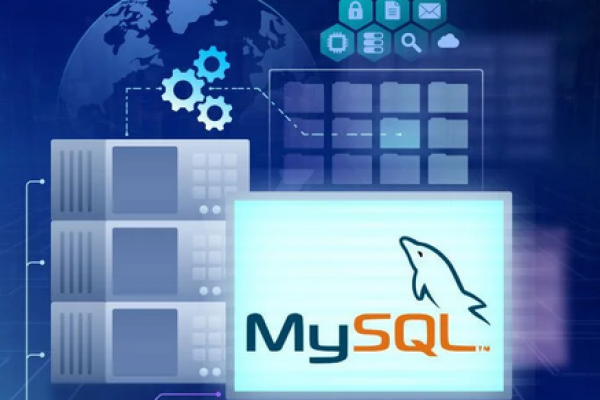
Discover why MySQL is perfect for large-scale projects with its scalability, security, cost-efficien...
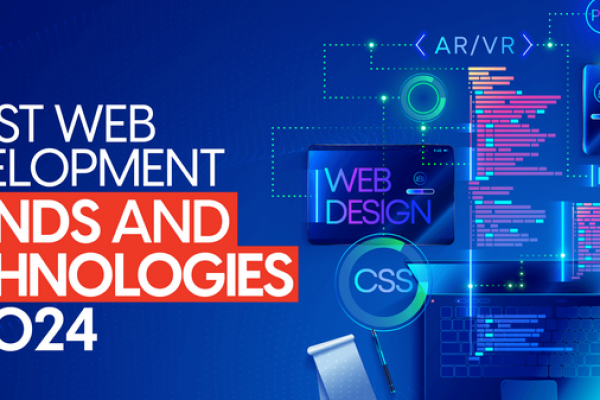
A look at the latest trends in web development for 2024, including new technologies, best practices,...
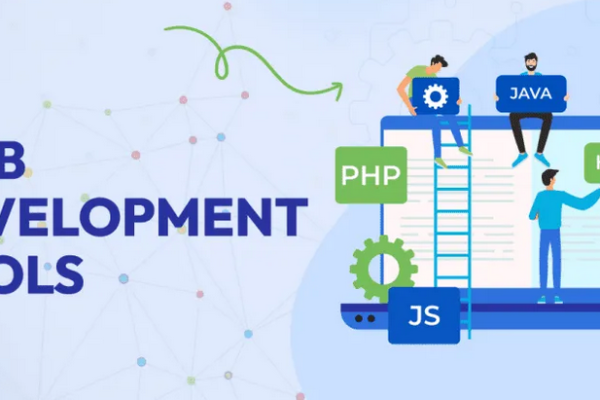
Discover the top 5 tools that are essential for web developers in 2024, from frameworks and librarie...